The Sieve of Hotchkiss offers an efficient way to find prime numbers by leveraging a key property: all prime numbers greater than 3 can be expressed in one of the forms 6k ± 1. This allows us to directly focus our search on these specific forms, significantly reducing the number of potential candidates that need to be checked.
The Sieve of Hotchkiss utilizes this property to create a unique sieving process that efficiently eliminates composite numbers. By focusing on the 6k ± 1 forms and systematically marking their multiples as composite, it can pinpoint prime numbers within these forms much faster than traditional methods.
Comparisons to Other Sieves:
Sieve of Eratosthenes: The Sieve of Eratosthenes checks all numbers up to the limit, while the Sieve of Hotchkiss focuses only on 6k ± 1 forms, significantly reducing the search space.
Sieve of Atkin: The Sieve of Atkin has a proven time complexity of O(n / log log n) and can find all primes up to a limit. However, for specific applications requiring only primes in the 6k ± 1 forms, the Sieve of Hotchkiss might be more efficient.
Reduced Search Space: The 6k ± 1 forms are the only forms that prime numbers greater than 3 can take. By focusing solely on these forms, the Sieve of Hotchkiss significantly reduces the search space compared to more general sieves that need to check all numbers up to a certain limit. This inherent efficiency stems directly from the algorithm’s focus on the most fundamental property of prime numbers.
Elimination of Unnecessary Calculations: By excluding all numbers that are not of the form 6k ± 1, the Sieve of Hotchkiss avoids checking many numbers that are obviously composite (multiples of 2 and 3). This directly translates to a reduction in calculations and improved speed.
Optimized Composite Marking: The algorithm also optimizes composite marking. Since we only need to consider the multiples of primes found within the 6k ± 1 forms, it’s more efficient in eliminating composite numbers compared to methods that need to check multiples of all primes found.
Direct Primality Check: The Sieve of Hotchkiss directly checks the primality of numbers within the 6k ± 1 forms, without requiring complex patterns or additional calculations. This streamlined approach contributes to its efficiency.
In essence, the Sieve of Hotchkiss is inherently efficient on the dimension of the search space and the number of calculations required. It leverages the fundamental properties of prime numbers and focuses directly on the most relevant forms, reducing redundancy and maximizing speed.
Optimization Considerations:
Prime Density: The density of prime numbers in the 6k ± 1 forms can be further explored to optimize the algorithm. You might be able to predict areas where primes are more likely to exist and focus the search in those regions.
Parallel Processing: The Sieve of Hotchkiss could be parallelized, potentially achieving significant speedups on multi-core processors.
Conclusion:
The Sieve of Hotchkiss is a useful tool for prime number generation, especially when focusing on primes within the 6k ± 1 forms. It offers a unique combination of efficiency, simplicity, and accuracy, particularly for applications where those forms are central. Further research into the optimization and potential extensions of the sieve could reveal its full power and lead to exciting new applications.
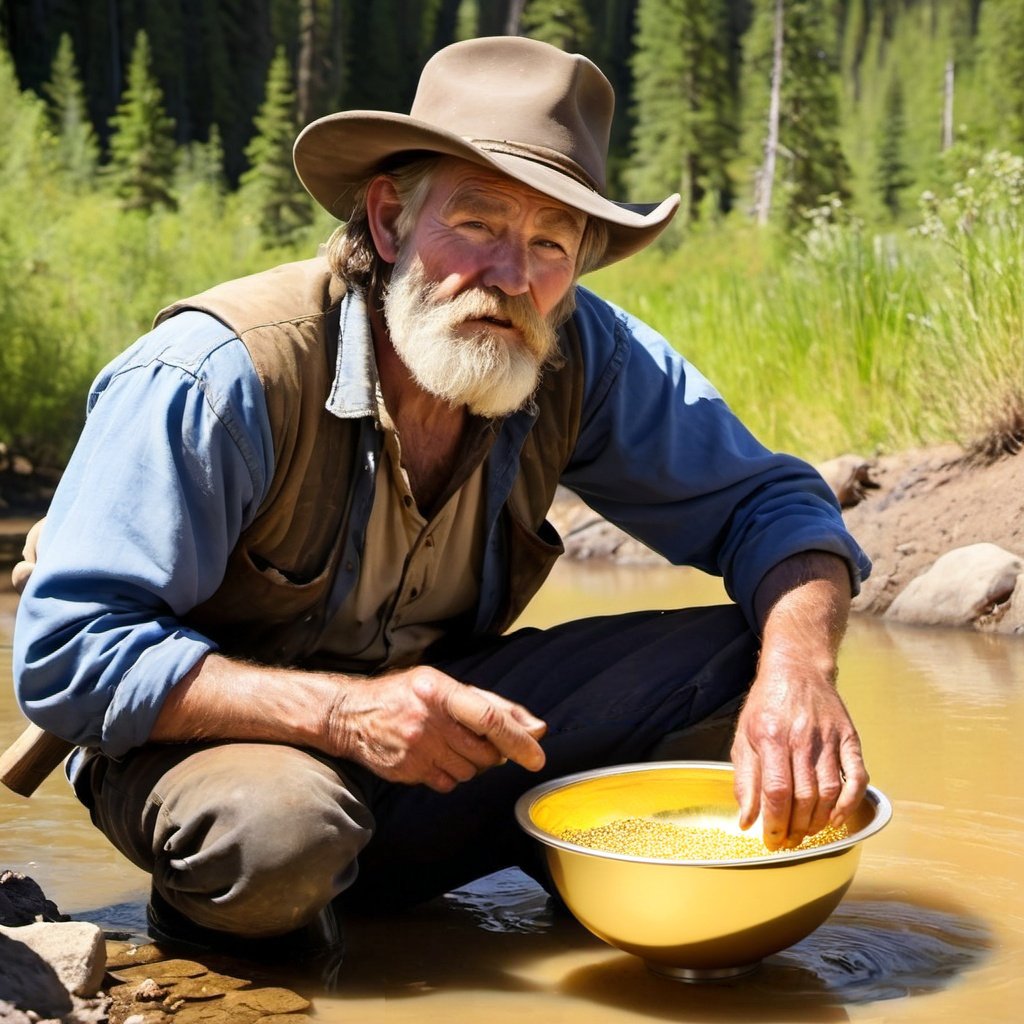
Sample Python Implementation which labels primes as A or B:
def sieve_of_hotchkiss(n):
"""
Implements the Sieve of Hotchkiss to find prime numbers
within the 6k ± 1 forms up to a given limit n,
efficiently handling composite exclusion and labeling them as A or B.
Args:
n: The upper limit for finding primes.
Returns:
A tuple containing the total number of primes found,
a list of prime numbers in form A (6k – 1),
a list of prime numbers in form B (6k + 1),
and a combined list of all prime numbers found.
"""
if n < 2:
return 0, [], [], []
# Initialize with 2 and 3
primes_a = [2]
primes_b = [3]
primes_combined = [(2, "A"), (3, "B")] if n >= 3 else [(2, "A")] if n >= 2 else []
# Boolean array to mark composites
is_composite = [False] * (n + 1)
# Check numbers of the form 6k ± 1
k = 1
while True:
p1 = 6 * k - 1
p2 = 6 * k + 1
if p1 > n:
break
# Check if 6k – 1 is a prime
if p1 <= n and not is_composite[p1]:
primes_a.append(p1)
primes_combined.append((p1, 'A'))
for multiple in range(p1 * p1, n + 1, p1):
is_composite[multiple] = True
# Check if 6k + 1 is a prime
if p2 <= n and not is_composite[p2]:
primes_b.append(p2)
primes_combined.append((p2, 'B'))
for multiple in range(p2 * p2, n + 1, p2):
is_composite[multiple] = True
k += 1
total_primes = len(primes_combined)
return total_primes, primes_a, primes_b, primes_combined
# Example usage:
n = 100
total, primes_a, primes_b, primes_combined = sieve_of_hotchkiss(n)
print(f"Total primes up to {n}: {total}")
print(f"Primes in form A: {primes_a}")
print(f"Primes in form B: {primes_b}")
print(f"All primes: {primes_combined}")